Generate Images with Google's Gemini API: A Node.js Application
Generate Images with Google's Gemini API: A Node.js Application
AI image generation is revolutionizing creative workflows. This blog post details the development of a Node.js application, the Gemini Image Generator, that harnesses Google's Gemini API to produce images from text prompts. We'll cover the technical implementation, API endpoints, and considerations for building a robust and efficient image generation service.
Project Overview
The Gemini Image Generator is a lightweight REST API enabling users to submit text prompts and receive AI-generated images. Built using Node.js, Express.js, and Google's Generative AI SDK, it offers a streamlined solution for image creation.
Key Features:
- Accepts text prompts for image generation.
- Utilizes Google's Gemini API for AI-powered image synthesis.
- Saves generated images on the server (consider cloud storage for scalability).
- Provides REST API endpoints for seamless integration with other applications.
Tech Stack
- Node.js: Backend runtime environment.
- Express.js: Lightweight and flexible web framework.
- Google Generative AI SDK: Facilitates interaction with the Gemini API.
- dotenv: Manages environment variables for secure API key handling.
- cors: Enables cross-origin resource sharing for broader application integration.
Getting Started
1. Clone the Repository
git clone https://github.com/manthanank/gemini-image-generator.git
cd gemini-image-generator
2. Install Dependencies
npm install
3. Configure Environment Variables
Create a .env
file in the project's root directory and add your Google Gemini API key:
GEMINI_API_KEY=your_google_gemini_api_key
PORT=5000
4. Start the Server
npm start
The server will be accessible at http://localhost:5000
.
API Endpoints
Generate an Image
Endpoint: POST /api/image/generate
Request Body:
{
"prompt": "a futuristic cityscape with neon lights"
}
Response:
{
"message": "Image generated successfully",
"imagePath": "temp/generated_image.png"
}
Project Structure
gemini-image-generator/
├── controllers/ // Business logic
├── routes/ // API routes
├── services/ // Google Gemini AI logic
├── temp/ // Generated images
├── server.js // Entry point
├── package.json // Dependencies
└── .env // Environment variables
Core Implementation
1. Setting Up the Express Server
The server.js
file initializes the Express application and starts the server:
const app = require("./app");
const { port } = require("./config/env");
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
2. Handling Image Generation Requests
The imageController.js
file handles incoming requests, validating the prompt and calling the Gemini API service:
const { generateImage } = require("../services/geminiService");
async function generateImageController(req, res) {
const { prompt } = req.body;
if (!prompt) {
return res.status(400).json({ error: "Prompt is required" });
}
try {
const imagePath = await generateImage(prompt);
res.status(200).json({ message: "Image generated successfully", imagePath });
} catch (error) {
res.status(500).json({ error: error.message });
}
}
module.exports = { generateImageController };
3. Integrating the Gemini API
The geminiService.js
file interacts with the Google Gemini API:
const { GoogleGenerativeAI } = require("@google/generative-ai");
const fs = require("fs");
const path = require("path");
const { geminiApiKey } = require("../config/env");
const genAI = new GoogleGenerativeAI(geminiApiKey);
async function generateImage(prompt) {
const model = genAI.getGenerativeModel({
model: "gemini-2.0-flash-exp-image-generation",
generationConfig: { responseModalities: ['Text', 'Image'] }
});
try {
const response = await model.generateContent(prompt);
for (const part of response.response.candidates[0].content.parts) {
if (part.inlineData) {
const imageData = part.inlineData.data;
const buffer = Buffer.from(imageData, 'base64');
const filePath = path.join(__dirname, '../temp/generated_image.png');
fs.writeFileSync(filePath, buffer);
return filePath;
}
}
} catch (error) {
console.error("Error generating image:", error);
throw new Error("Failed to generate image");
}
}
module.exports = { generateImage };
Conclusion
This project demonstrates a practical implementation of Google's Gemini API for image generation. Further development could incorporate features like image style selection, real-time previews, and integration with cloud storage for enhanced scalability and reliability. Remember to always handle API keys securely and consider error handling and rate limiting for a production-ready application.
Related Articles
Software Development
Unveiling the Haiku License: A Fair Code Revolution
Dive into the innovative Haiku License, a game-changer in open-source licensing that balances open access with fair compensation for developers. Learn about its features, challenges, and potential to reshape the software development landscape. Explore now!
Read MoreSoftware Development
Leetcode - 1. Two Sum
Master LeetCode's Two Sum problem! Learn two efficient JavaScript solutions: the optimal hash map approach and a practical two-pointer technique. Improve your coding skills today!
Read MoreBusiness, Software Development
The Future of Digital Credentials in 2025: Trends, Challenges, and Opportunities
Digital credentials are transforming industries in 2025! Learn about blockchain's role, industry adoption trends, privacy enhancements, and the challenges and opportunities shaping this exciting field. Discover how AI and emerging technologies are revolutionizing identity verification and workforce management. Explore the future of digital credentials today!
Read MoreSoftware Development
Unlocking the Secrets of AWS Pricing: A Comprehensive Guide
Master AWS pricing with this comprehensive guide! Learn about various pricing models, key cost factors, and practical tips for optimizing your cloud spending. Unlock significant savings and efficiently manage your AWS infrastructure.
Read MoreSoftware Development
Exploring the GNU Verbatim Copying License
Dive into the GNU Verbatim Copying License (GVCL): Understand its strengths, weaknesses, and impact on open-source collaboration. Explore its unique approach to code integrity and its relevance in today's software development landscape. Learn more!
Read MoreSoftware Development
Unveiling the FSF Unlimited License: A Fairer Future for Open Source?
Explore the FSF Unlimited License: a groundbreaking open-source license designed to balance free software distribution with fair developer compensation. Learn about its origins, strengths, limitations, and real-world impact. Discover how it addresses the challenges of open-source sustainability and innovation.
Read MoreSoftware Development
Conquer JavaScript in 2025: A Comprehensive Learning Roadmap
Master JavaScript in 2025! This comprehensive roadmap guides you through fundamental concepts, modern frameworks like React, and essential tools. Level up your skills and build amazing web applications – start learning today!
Read MoreBusiness, Software Development
Building a Successful Online Gambling Website: A Comprehensive Guide
Learn how to build a successful online gambling website. This comprehensive guide covers key considerations, technical steps, essential tools, and best practices for creating a secure and engaging platform. Start building your online gambling empire today!
Read MoreSoftware Development
Discover Ocak.co: Your Premier Online Forum
Explore Ocak.co, a vibrant online forum connecting people through shared interests. Engage in discussions, share ideas, and find answers. Join the conversation today!
Read MoreSoftware Development
Mastering URL Functions in Presto/Athena
Unlock the power of Presto/Athena's URL functions! Learn how to extract hostnames, parameters, paths, and more from URLs for efficient data analysis. Master these essential functions for web data processing today!
Read MoreSoftware Development
Introducing URL Opener: Open Multiple URLs Simultaneously
Tired of opening multiple URLs one by one? URL Opener lets you open dozens of links simultaneously with one click. Boost your productivity for SEO, web development, research, and more! Try it now!
Read More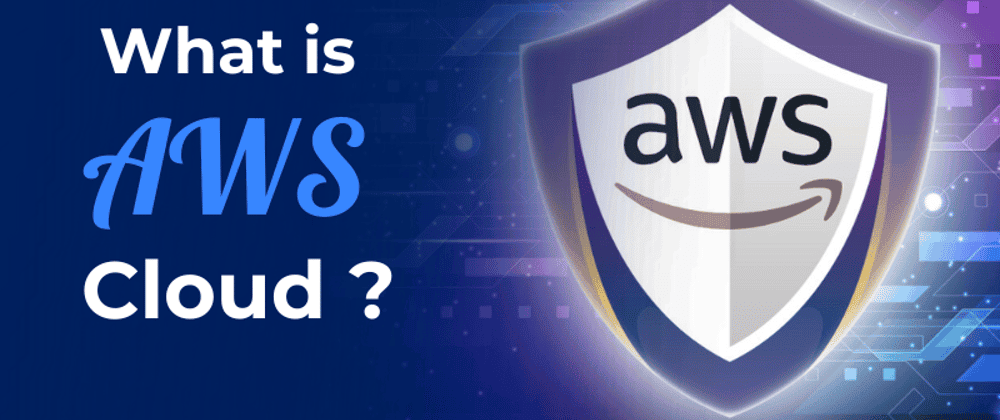
Software Development, Business
Unlocking the Power of AWS: A Deep Dive into Amazon Web Services
Dive deep into Amazon Web Services (AWS)! This comprehensive guide explores key features, benefits, and use cases, empowering businesses of all sizes to leverage cloud computing effectively. Learn about scalability, cost-effectiveness, and global infrastructure. Start your AWS journey today!
Read MoreSoftware Development
Understanding DNS in Kubernetes with CoreDNS
Master CoreDNS in Kubernetes: This guide unravels the complexities of CoreDNS, Kubernetes's default DNS server, covering configuration, troubleshooting, and optimization for seamless cluster performance. Learn best practices and avoid common pitfalls!
Read MoreSoftware Development
EUPL 1.1: A Comprehensive Guide to Fair Open Source Licensing
Dive into the EUPL 1.1 open-source license: understand its strengths, challenges, and real-world applications for fair code. Learn how it balances freedom and developer protection. Explore now!
Read MoreSoftware Development
Erlang Public License 1.1: Open Source Protection Deep Dive
Dive deep into the Erlang Public License 1.1 (EPL 1.1), a crucial open-source license balancing collaboration and contributor protection. Learn about its strengths, challenges, and implications for developers and legal teams.
Read MoreSoftware Development
Unlocking Kerala's IT Job Market: Your Path to Data Science Success
Launch your data science career in Kerala's booming IT sector! Learn the in-demand skills to land high-paying jobs. Discover top data science courses & career paths. Enroll today!
Read More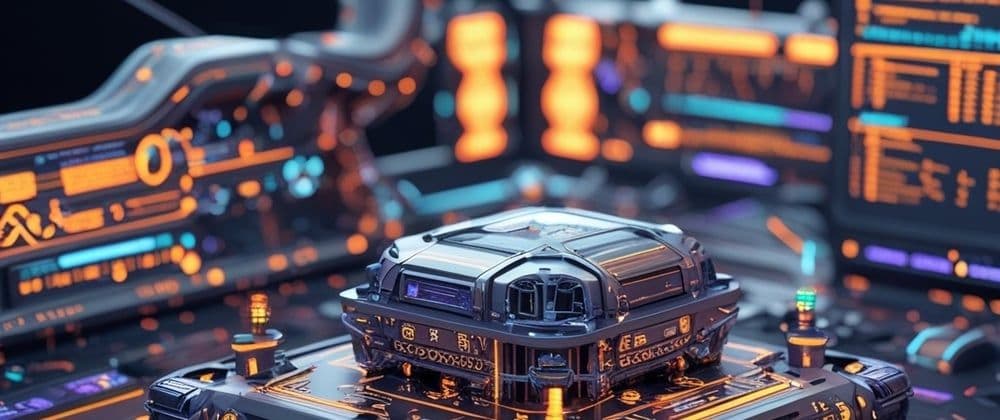
Software Development
Automation in Software Testing: A Productivity Booster
Supercharge your software testing with automation! Learn how to boost productivity, efficiency, and accuracy using automation tools and best practices. Discover real-world examples and get started today!
Read MoreSoftware Development
Mastering Anagram Grouping in JavaScript
Master efficient anagram grouping in JavaScript! Learn two proven methods: sorting and character counting. Optimize your code for speed and explore key JavaScript concepts like charCodeAt(). Improve your algorithms today!
Read More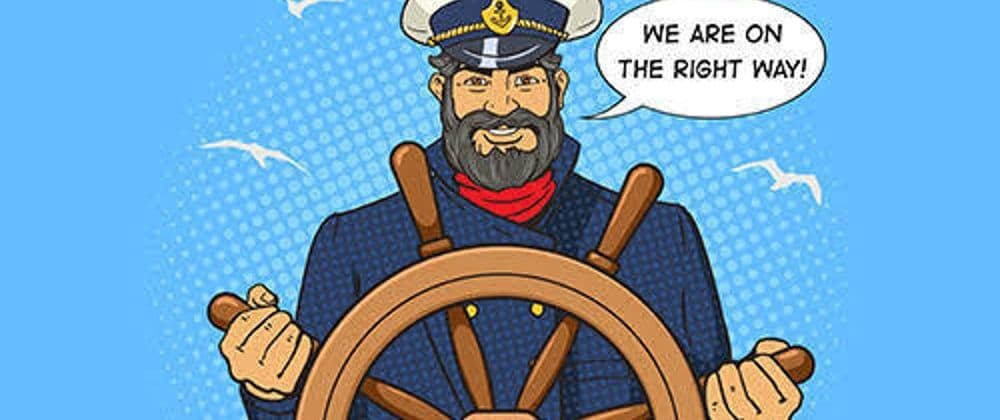
Software Development
Mastering Kubernetes Deployments: Rolling Updates and Scaling
Master Kubernetes Deployments for seamless updates & scaling. Learn rolling updates, autoscaling, and best practices for high availability and efficient resource use. Improve your application management today!
Read More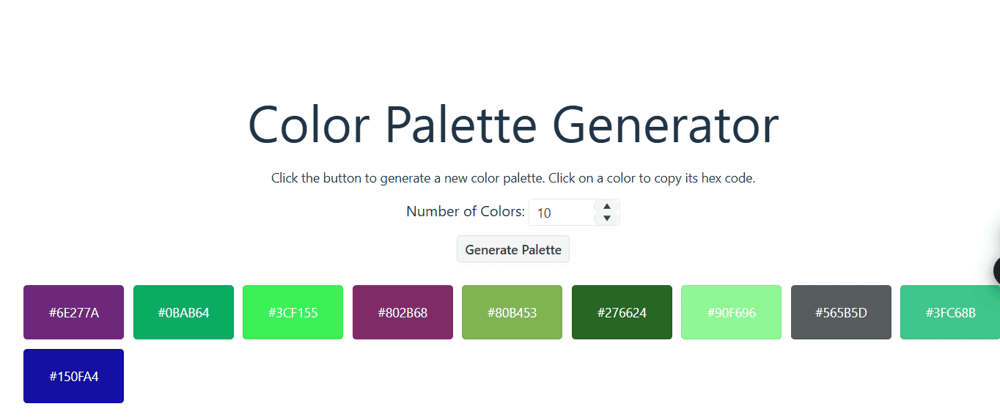
Software Development
KendoReact Color Palette Generator: A Powerful, Free Component Showcase
Generate stunning color palettes effortlessly with this React app built using free KendoReact components. Customize the number of colors, copy hex codes, and enjoy smooth animations. Build your perfect palette today!
Read More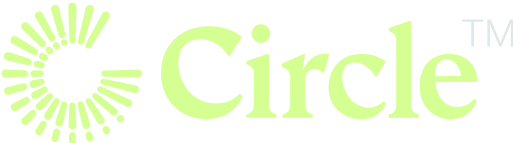