Leetcode - 1. Two Sum
Leetcode - 1. Two Sum: Efficient Solutions in JavaScript
Finding pairs of numbers within an array that sum to a specified target is a classic coding interview problem. This post explores two efficient approaches to solve LeetCode's "Two Sum" problem using JavaScript: one leveraging the power of hash maps, and another employing a two-pointer technique.
Using a Hash Map (Optimal Solution)
A hash map (or dictionary in Python) provides an optimal solution for this problem due to its constant-time average complexity for lookups. This approach minimizes the time it takes to find the solution, especially when working with large datasets.
/**
* @param {number[]} nums
* @param {number} target
* @return {number[]}
*/
var twoSum = function (nums, target) {
let myMap = new Map();
for (let i = 0; i < nums.length; i++) {
myMap.set(nums[i], i);
}
for (let i = 0; i < nums.length; i++) {
let remaining = target - nums[i];
if (myMap.has(remaining) && myMap.get(remaining) !== i) {
return [i, myMap.get(remaining)];
}
}
};
This code first populates a hash map with each number in the input array and its index. Then, it iterates through the array, calculating the 'remaining' value needed to reach the target. By checking if the 'remaining' value exists in the map, and ensuring it's not the same index, we efficiently locate the pair.
Example: Given nums = [2, 7, 11, 15]
and target = 9
, the function would return [0, 1]
because nums[0] + nums[1] = 2 + 7 = 9
.
Using Pointers (Alternative Approach)
While the hash map offers optimal performance, a two-pointer approach provides an alternative solution, particularly useful when the input array is already sorted. This method trades off some performance for a potentially simpler implementation.
/**
* @param {number[]} nums
* @param {number} target
* @return {number[]}
*/
var twoSum = function(nums, target) {
let left = 0;
let right = 1
while(left <= nums.length-1){
if(nums[left] + nums[right] === target){
return [left,right]
}else if (right === nums.length-1){
left++;
right = left+1;
}
else{
right++;
}
}
};
Note: This two-pointer approach assumes the input array nums
is sorted. If it's not sorted, you'll need to sort it first, adding to the overall time complexity.
Example: Given a sorted nums = [2, 7, 11, 15]
and target = 9
, the function would efficiently find and return [0, 1]
.
Conclusion
Both hash map and two-pointer approaches effectively solve the Two Sum problem. The hash map offers superior average-case time complexity (O(n)), making it ideal for larger datasets. The two-pointer method, efficient for sorted arrays, presents a concise alternative. Choosing the best approach depends on the specific constraints and characteristics of your input data.
Related Articles
Software Development
Unveiling the Haiku License: A Fair Code Revolution
Dive into the innovative Haiku License, a game-changer in open-source licensing that balances open access with fair compensation for developers. Learn about its features, challenges, and potential to reshape the software development landscape. Explore now!
Read MoreBusiness, Software Development
The Future of Digital Credentials in 2025: Trends, Challenges, and Opportunities
Digital credentials are transforming industries in 2025! Learn about blockchain's role, industry adoption trends, privacy enhancements, and the challenges and opportunities shaping this exciting field. Discover how AI and emerging technologies are revolutionizing identity verification and workforce management. Explore the future of digital credentials today!
Read MoreSoftware Development
Unlocking the Secrets of AWS Pricing: A Comprehensive Guide
Master AWS pricing with this comprehensive guide! Learn about various pricing models, key cost factors, and practical tips for optimizing your cloud spending. Unlock significant savings and efficiently manage your AWS infrastructure.
Read MoreSoftware Development
Exploring the GNU Verbatim Copying License
Dive into the GNU Verbatim Copying License (GVCL): Understand its strengths, weaknesses, and impact on open-source collaboration. Explore its unique approach to code integrity and its relevance in today's software development landscape. Learn more!
Read MoreSoftware Development
Unveiling the FSF Unlimited License: A Fairer Future for Open Source?
Explore the FSF Unlimited License: a groundbreaking open-source license designed to balance free software distribution with fair developer compensation. Learn about its origins, strengths, limitations, and real-world impact. Discover how it addresses the challenges of open-source sustainability and innovation.
Read MoreSoftware Development
Conquer JavaScript in 2025: A Comprehensive Learning Roadmap
Master JavaScript in 2025! This comprehensive roadmap guides you through fundamental concepts, modern frameworks like React, and essential tools. Level up your skills and build amazing web applications – start learning today!
Read MoreBusiness, Software Development
Building a Successful Online Gambling Website: A Comprehensive Guide
Learn how to build a successful online gambling website. This comprehensive guide covers key considerations, technical steps, essential tools, and best practices for creating a secure and engaging platform. Start building your online gambling empire today!
Read MoreAI, Software Development
Generate Images with Google's Gemini API: A Node.js Application
Learn how to build an AI-powered image generator using Google's Gemini API and Node.js. This comprehensive guide covers setup, API integration, and best practices for creating a robust image generation service. Start building today!
Read MoreSoftware Development
Discover Ocak.co: Your Premier Online Forum
Explore Ocak.co, a vibrant online forum connecting people through shared interests. Engage in discussions, share ideas, and find answers. Join the conversation today!
Read MoreSoftware Development
Mastering URL Functions in Presto/Athena
Unlock the power of Presto/Athena's URL functions! Learn how to extract hostnames, parameters, paths, and more from URLs for efficient data analysis. Master these essential functions for web data processing today!
Read MoreSoftware Development
Introducing URL Opener: Open Multiple URLs Simultaneously
Tired of opening multiple URLs one by one? URL Opener lets you open dozens of links simultaneously with one click. Boost your productivity for SEO, web development, research, and more! Try it now!
Read More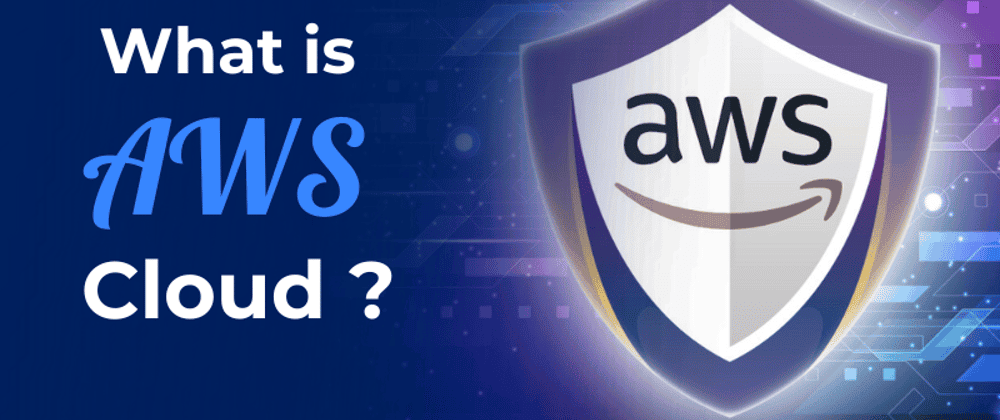
Software Development, Business
Unlocking the Power of AWS: A Deep Dive into Amazon Web Services
Dive deep into Amazon Web Services (AWS)! This comprehensive guide explores key features, benefits, and use cases, empowering businesses of all sizes to leverage cloud computing effectively. Learn about scalability, cost-effectiveness, and global infrastructure. Start your AWS journey today!
Read MoreSoftware Development
Understanding DNS in Kubernetes with CoreDNS
Master CoreDNS in Kubernetes: This guide unravels the complexities of CoreDNS, Kubernetes's default DNS server, covering configuration, troubleshooting, and optimization for seamless cluster performance. Learn best practices and avoid common pitfalls!
Read MoreSoftware Development
EUPL 1.1: A Comprehensive Guide to Fair Open Source Licensing
Dive into the EUPL 1.1 open-source license: understand its strengths, challenges, and real-world applications for fair code. Learn how it balances freedom and developer protection. Explore now!
Read MoreSoftware Development
Erlang Public License 1.1: Open Source Protection Deep Dive
Dive deep into the Erlang Public License 1.1 (EPL 1.1), a crucial open-source license balancing collaboration and contributor protection. Learn about its strengths, challenges, and implications for developers and legal teams.
Read MoreSoftware Development
Unlocking Kerala's IT Job Market: Your Path to Data Science Success
Launch your data science career in Kerala's booming IT sector! Learn the in-demand skills to land high-paying jobs. Discover top data science courses & career paths. Enroll today!
Read More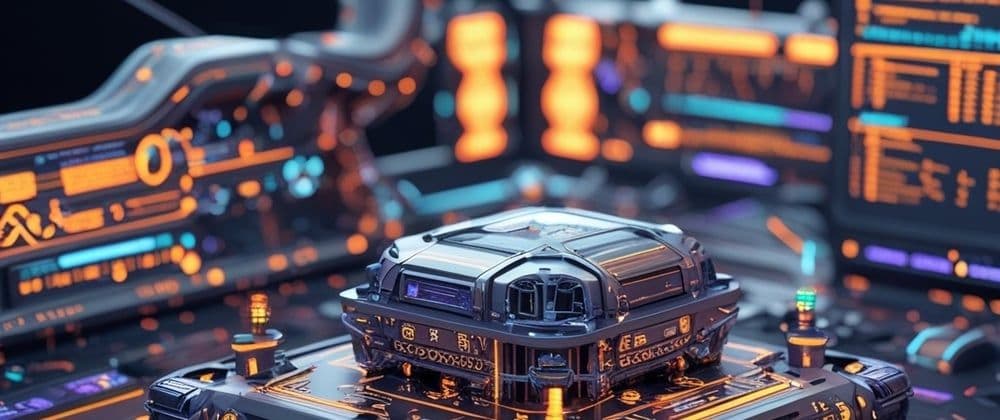
Software Development
Automation in Software Testing: A Productivity Booster
Supercharge your software testing with automation! Learn how to boost productivity, efficiency, and accuracy using automation tools and best practices. Discover real-world examples and get started today!
Read MoreSoftware Development
Mastering Anagram Grouping in JavaScript
Master efficient anagram grouping in JavaScript! Learn two proven methods: sorting and character counting. Optimize your code for speed and explore key JavaScript concepts like charCodeAt(). Improve your algorithms today!
Read More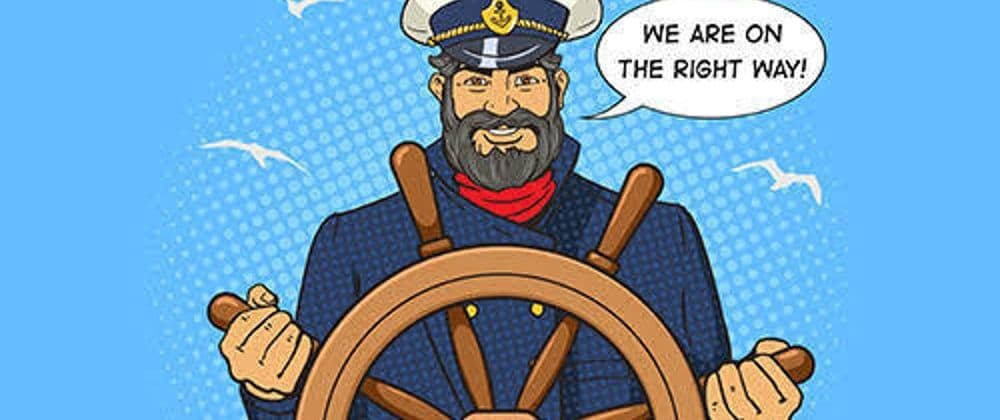
Software Development
Mastering Kubernetes Deployments: Rolling Updates and Scaling
Master Kubernetes Deployments for seamless updates & scaling. Learn rolling updates, autoscaling, and best practices for high availability and efficient resource use. Improve your application management today!
Read More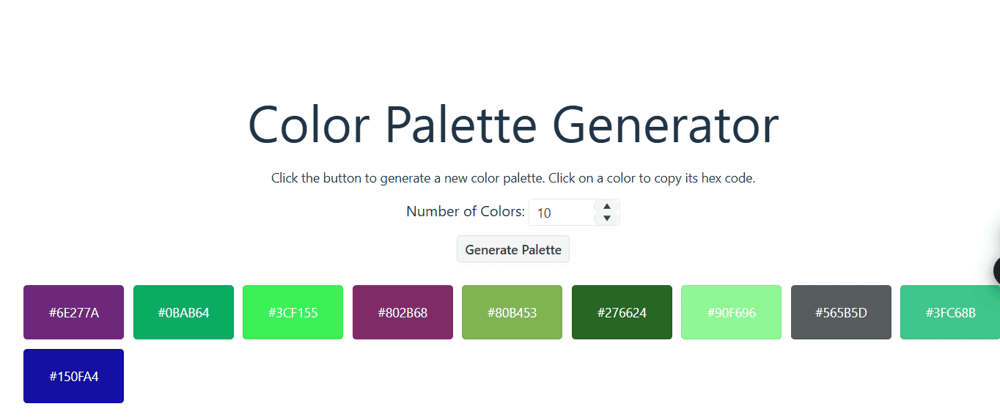
Software Development
KendoReact Color Palette Generator: A Powerful, Free Component Showcase
Generate stunning color palettes effortlessly with this React app built using free KendoReact components. Customize the number of colors, copy hex codes, and enjoy smooth animations. Build your perfect palette today!
Read More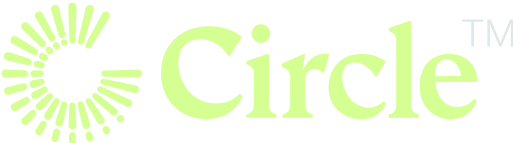