Mastering Anagram Grouping in JavaScript
Efficiently Grouping Anagrams in JavaScript
Anagrams are words or phrases formed by rearranging the letters of another word or phrase. This seemingly simple concept presents a fun algorithmic challenge: given an array of strings, how can we efficiently group together all the anagrams? This post explores two approaches to solve this problem in JavaScript, comparing their time complexities and providing detailed explanations.
Method 1: Sorting for Anagram Identification
Our first approach leverages the fundamental property of anagrams: they have the same letters, just in a different order. If we sort the letters of each word, anagrams will have identical sorted forms. This insight allows us to use a Map
to group anagrams efficiently.
/**
* @param {string[]} strs
* @return {string[][]}
*/
var groupAnagrams = function(strs) {
let res = new Map();
for (let s of strs) {
const key = s.split('').sort().join('');
if (!res.has(key)) {
res.set(key, []);
}
res.get(key).push(s);
}
return Array.from(res.values());
};
This method has a time complexity of O(n * m log m), where n
is the number of strings and m
is the maximum length of a string. The dominant factor is the sorting step (m log m
) for each string.
Method 2: Character Counting for Optimized Anagram Detection
While sorting is straightforward, we can achieve better performance using character counting. Instead of sorting, we create a frequency array representing the count of each character (a-z) in a string. Anagrams will share the same character frequency array. This leads to a more optimized solution.
/**
* @param {string[]} strs
* @return {string[][]}
*/
var groupAnagrams = function(strs) {
let res = new Map();
for (let s of strs) {
const count = new Array(26).fill(0);
for (c of s) {
count[c.charCodeAt(0) - "a".charCodeAt(0)] += 1;
}
const key = count.join(',');
if (!res.has(key)) {
res.set(key, []);
}
res.get(key).push(s);
}
return Array.from(res.values());
};
This character counting approach boasts a time complexity of O(n * m), significantly faster than the sorting method for larger input sets, as it avoids the logarithmic overhead of sorting.
Understanding Key JavaScript Concepts
charCodeAt(index)
This method is crucial in the character counting method. It returns the Unicode value of the character at a given index. For example, "a".charCodeAt(0)
returns 97, the Unicode value of 'a'. This allows us to efficiently index into our frequency array.
res.get(key).push(s);
This line retrieves the array associated with the key in the Map
and efficiently pushes the current string s
onto it. Because arrays in JavaScript are reference types, the Map
is updated in place; there's no need for a separate set
operation.
Array.from(res.values())
The res.values()
method returns an iterator of the values (arrays of anagrams) in the Map
. Array.from()
converts this iterator into a standard array, fulfilling the function's requirement of returning a string[][]
.
Conclusion
We've explored two distinct methods for grouping anagrams in JavaScript. The character counting method offers superior performance due to its linear time complexity, making it the preferred choice for larger datasets. Understanding the underlying principles of character encoding and JavaScript's data structures is key to crafting efficient and elegant solutions to this classic algorithmic problem. Try implementing these methods and experimenting with various input sizes to observe the performance differences firsthand!
Related Articles
Software Development
Unveiling the Haiku License: A Fair Code Revolution
Dive into the innovative Haiku License, a game-changer in open-source licensing that balances open access with fair compensation for developers. Learn about its features, challenges, and potential to reshape the software development landscape. Explore now!
Read MoreSoftware Development
Leetcode - 1. Two Sum
Master LeetCode's Two Sum problem! Learn two efficient JavaScript solutions: the optimal hash map approach and a practical two-pointer technique. Improve your coding skills today!
Read MoreBusiness, Software Development
The Future of Digital Credentials in 2025: Trends, Challenges, and Opportunities
Digital credentials are transforming industries in 2025! Learn about blockchain's role, industry adoption trends, privacy enhancements, and the challenges and opportunities shaping this exciting field. Discover how AI and emerging technologies are revolutionizing identity verification and workforce management. Explore the future of digital credentials today!
Read MoreSoftware Development
Unlocking the Secrets of AWS Pricing: A Comprehensive Guide
Master AWS pricing with this comprehensive guide! Learn about various pricing models, key cost factors, and practical tips for optimizing your cloud spending. Unlock significant savings and efficiently manage your AWS infrastructure.
Read MoreSoftware Development
Exploring the GNU Verbatim Copying License
Dive into the GNU Verbatim Copying License (GVCL): Understand its strengths, weaknesses, and impact on open-source collaboration. Explore its unique approach to code integrity and its relevance in today's software development landscape. Learn more!
Read MoreSoftware Development
Unveiling the FSF Unlimited License: A Fairer Future for Open Source?
Explore the FSF Unlimited License: a groundbreaking open-source license designed to balance free software distribution with fair developer compensation. Learn about its origins, strengths, limitations, and real-world impact. Discover how it addresses the challenges of open-source sustainability and innovation.
Read MoreSoftware Development
Conquer JavaScript in 2025: A Comprehensive Learning Roadmap
Master JavaScript in 2025! This comprehensive roadmap guides you through fundamental concepts, modern frameworks like React, and essential tools. Level up your skills and build amazing web applications – start learning today!
Read MoreBusiness, Software Development
Building a Successful Online Gambling Website: A Comprehensive Guide
Learn how to build a successful online gambling website. This comprehensive guide covers key considerations, technical steps, essential tools, and best practices for creating a secure and engaging platform. Start building your online gambling empire today!
Read MoreAI, Software Development
Generate Images with Google's Gemini API: A Node.js Application
Learn how to build an AI-powered image generator using Google's Gemini API and Node.js. This comprehensive guide covers setup, API integration, and best practices for creating a robust image generation service. Start building today!
Read MoreSoftware Development
Discover Ocak.co: Your Premier Online Forum
Explore Ocak.co, a vibrant online forum connecting people through shared interests. Engage in discussions, share ideas, and find answers. Join the conversation today!
Read MoreSoftware Development
Mastering URL Functions in Presto/Athena
Unlock the power of Presto/Athena's URL functions! Learn how to extract hostnames, parameters, paths, and more from URLs for efficient data analysis. Master these essential functions for web data processing today!
Read MoreSoftware Development
Introducing URL Opener: Open Multiple URLs Simultaneously
Tired of opening multiple URLs one by one? URL Opener lets you open dozens of links simultaneously with one click. Boost your productivity for SEO, web development, research, and more! Try it now!
Read More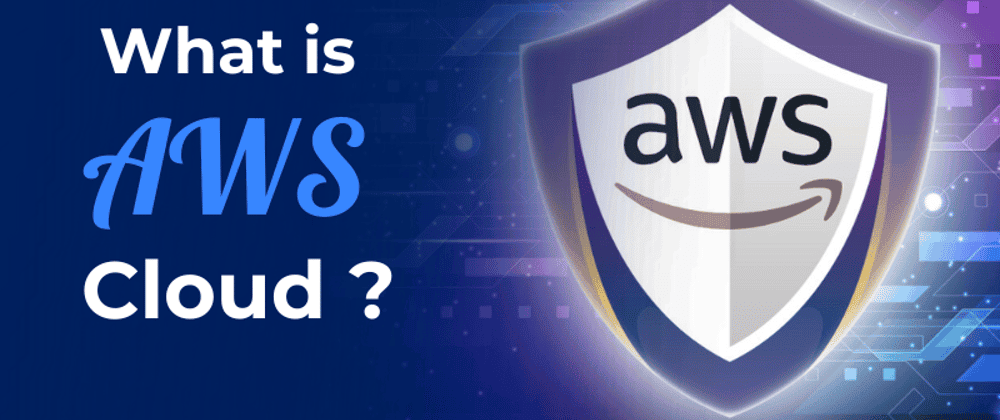
Software Development, Business
Unlocking the Power of AWS: A Deep Dive into Amazon Web Services
Dive deep into Amazon Web Services (AWS)! This comprehensive guide explores key features, benefits, and use cases, empowering businesses of all sizes to leverage cloud computing effectively. Learn about scalability, cost-effectiveness, and global infrastructure. Start your AWS journey today!
Read MoreSoftware Development
Understanding DNS in Kubernetes with CoreDNS
Master CoreDNS in Kubernetes: This guide unravels the complexities of CoreDNS, Kubernetes's default DNS server, covering configuration, troubleshooting, and optimization for seamless cluster performance. Learn best practices and avoid common pitfalls!
Read MoreSoftware Development
EUPL 1.1: A Comprehensive Guide to Fair Open Source Licensing
Dive into the EUPL 1.1 open-source license: understand its strengths, challenges, and real-world applications for fair code. Learn how it balances freedom and developer protection. Explore now!
Read MoreSoftware Development
Erlang Public License 1.1: Open Source Protection Deep Dive
Dive deep into the Erlang Public License 1.1 (EPL 1.1), a crucial open-source license balancing collaboration and contributor protection. Learn about its strengths, challenges, and implications for developers and legal teams.
Read MoreSoftware Development
Unlocking Kerala's IT Job Market: Your Path to Data Science Success
Launch your data science career in Kerala's booming IT sector! Learn the in-demand skills to land high-paying jobs. Discover top data science courses & career paths. Enroll today!
Read More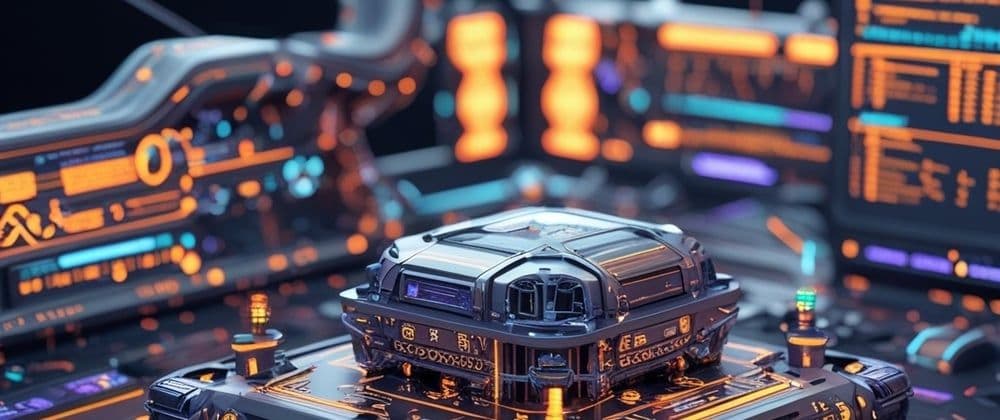
Software Development
Automation in Software Testing: A Productivity Booster
Supercharge your software testing with automation! Learn how to boost productivity, efficiency, and accuracy using automation tools and best practices. Discover real-world examples and get started today!
Read More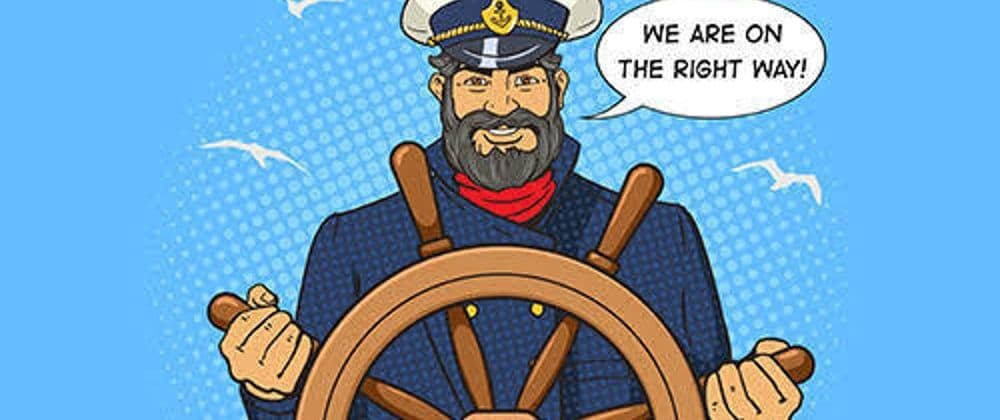
Software Development
Mastering Kubernetes Deployments: Rolling Updates and Scaling
Master Kubernetes Deployments for seamless updates & scaling. Learn rolling updates, autoscaling, and best practices for high availability and efficient resource use. Improve your application management today!
Read More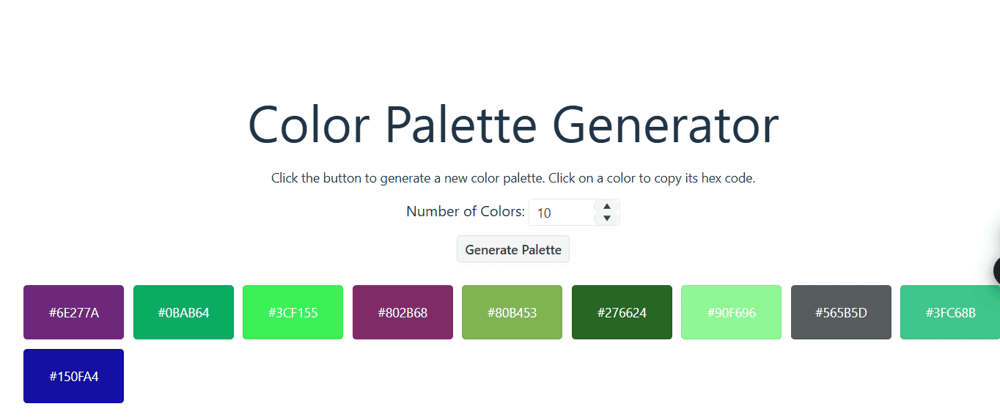
Software Development
KendoReact Color Palette Generator: A Powerful, Free Component Showcase
Generate stunning color palettes effortlessly with this React app built using free KendoReact components. Customize the number of colors, copy hex codes, and enjoy smooth animations. Build your perfect palette today!
Read More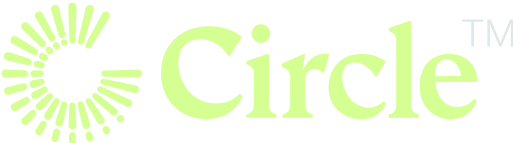